Hierarchical Multi Module Project
The previous tutorial explained the structure of a simple multi module project. Let’s extend it further to learn advanced features of Maven multi module project.
The example code is available at GitHub Maven Examples. Download the examples as zip and extract it to some location or clone it with git.
Flat vs Hierarchical Layout
In extended-multi project, we add one more module to simple-multi and
the new module, config
, has one class ConfigService that uses Apache
Commons Configuration library to manage configurations of the app.
We can keep on adding new modules to the top-level directory as we have done with app and util modules in simple-multi. But, with this flat layout, we soon end up with lots of module in the top-level directory.
Instead we can structure the project hierarchically, and group util
and config
modules under another module (for example named as shared)
and add the shared
module to top-level parent POM.
The flat and hierarchical layout is shown below (parent POM are highlighted with yellow)
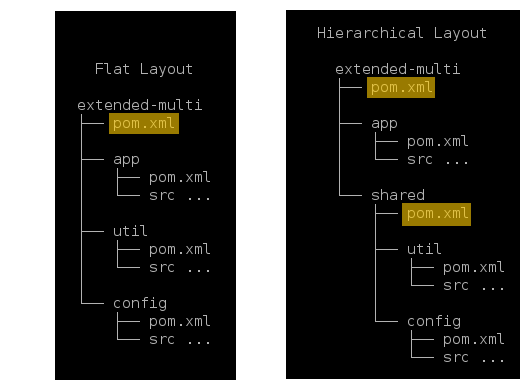
To build extended-multi, go to the extracted directory and run
$ cd extended-multi
$ mvn test
which builds shared
, util
, config
and app
modules in that order.
Commons Configurations
By the way, Apache Commons Configuration is quite useful to manage configurations from multiple sources such as XML, property file or system. But, for simplicity, in extended-multi we just hardcoded a config value and retrieve it via getter. In case, you like to use it any of your projects, then see our Gotz Project ConfigService class for complete example.
Example
Let’s go through the structure of extended-multi
project. The top
level directory contains parent POM and two module directories - app and
shared.
The parent POM specifies maven coordinates and packaging type as pom.
The modules/module
element adds app and shared modules.
extended-multi/pom.xml
....
<groupId>org.codetab</groupId>
<artifactId>extended-multi</artifactId>
<version>1.0</version>
<packaging>pom</packaging>
<modules>
<module>app</module>
<module>shared</module>
</modules>
....
There is no much change in app module which we saw in the previous tutorial expect that it specifies extended-multi as its parent.
The shared
module folder contains two module directories - util and
config and a parent POM. The contents of shared/pom.xml
is as below
....
<parent>
<groupId>org.codetab</groupId>
<artifactId>extended-multi</artifactId>
<version>1.0</version>
</parent>
<artifactId>shared</artifactId>
<packaging>pom</packaging>
<modules>
<module>util</module>
<module>config</module>
</modules>
....
It specifies that extended-multi
as its parent and adds two modules -
util
and config
. The packaging type is set as pom
as there is no
source directory in it.
The config module pom.xml specifies that shared module is its parent and uses commons configurations as dependency.
shared/config/pom.xml
....
<parent>
<groupId>org.codetab</groupId>
<artifactId>shared</artifactId>
<version>1.0</version>
</parent>
<artifactId>util</artifactId>
<dependencies>
....
<dependency>
<groupId>org.apache.commons</groupId>
<version>3.6</version>
<artifactId>commons-lang3</artifactId>
</dependency>
</dependencies>
....
Similarly, the shared/util/pom.xml
specifies that shared module is its
parent.
Build
To build maven multi module project, we have to run maven commands from the top level folder of the project. Maven processes top level POM and the module POMs and after all POMs are processed, the reactor properly sequences the modules and then builds each module.
When mvn test
command is run, Maven first runs test phase for
extended-multi but as there are no source files in top level project,
phase executes without doing anything and then it complies and tests
shared and finally, the app module.
For command mvn clean
, maven cleans all modules one by one.
When command mvn clean install
is run from the top level folder, maven
compiles, tests and installs all modules - extended-multi (just the
pom.xml), shared (just the pom.xml), util, config and app - to the local
repository.
In the next tutorial, we explain inheritance which is useful to compact the multi module POM.