6.1. WordPress Add Menu
Share on Social Plugin uses common options such as FaceBook App ID. In this section, we add a new menu item - Settings - to WordPress Administration Menu to display the option page.
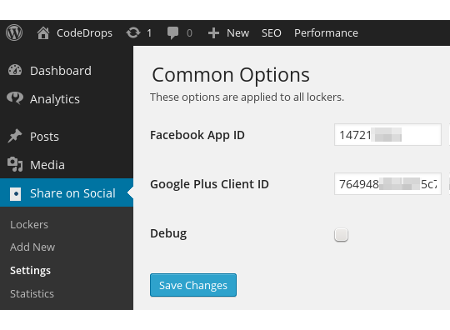
In Share on Social Plugin, class file admin/class-options.php
defines
class Sos_Options
to manage the Settings page.
share-on-social/admin/admin.php
require_once SOS_PATH . 'admin/class-options.php';
/**
* Class to load admin modules
*/
class Sos_Admin {
/**
* initializes the admin modules
*/
function setup () {
$sos_options = new Sos_Options();
$sos_options->setup();
...
We instantiate Sos_Options class in admin.php
and call its setup()
method. Let’s see what happens in Sos_Options::setup().
share-on-social/admin/class-options.php
class Sos_Options {
public function setup () {
add_action( 'admin_init',
array(
$this,
'init_common_options'
) );
add_action( 'admin_menu',
array(
$this,
'register_settings_page'
) );
}
....
As explained in the previous chapter - Hooks, Actions and Filters, we add actions to bind class methods to actions hooks.
add
init_common_options()
method as action function to action hook admin_init.add
register_settings_page()
method as action function to action hook admin_menu.
When we access admin screen, WordPress core after executing typical hooks such as plugins_loaded, init etc., encounters admin_menu hook and it will call Sos_Options::register_settings_page(). We use this to add a new menu item to admin menu.
Add Administration Menu item
The action functions of admin_menu hook are used to add new menu
item or sub menu item to WordPress Admin menu. In the function, we use
WordPress function add_submenu_page()
to add a new sub menu item and
link it to a menu page.
share-on-social/admin/class-options.php
public function register_settings_page () {
$page_title = __( 'Common Options', 'sos-domain' );
$menu_label = __( 'Sos Settings', 'sos-domain' );
$setting_page = 'sos_settings_page';
add_submenu_page('options-general.php', $page_title, $menu_label,
'administrator', $setting_page,
array($this,'render_settings_page') );
}
To define strings that are translatable to other languages for
internationalization, WordPress uses __() functions such as
$page_title = __( 'Common Options', 'sos-domain' )
. We will explain
this in detail in a later chapter and for the time being you may think
them as regular string assignment like $page_title = 'Common Options'
.
After defining variables, we call add_submenu_page() function with following parameters.
parent_slug - slug name of the menu to which this sub menu should attach. We attach our menu item to options-general.php which is slug name for Settings menu in the admin screen. Refer add_submenu_page() to find out the names of parent slugs that the admin menu uses.
page_title - title to use on the page linked to this menu.
menu_title - menu item label.
capability - minimum capability of user for whom this menu is displayed. As we wish show this menu only admin we set it to administrator.
menu_slug - slug name for this menu. We use sos_settings_page as slug name for this menu.
callback function - the call back function which renders the page attached to this menu. When menu is clicked, WordPress calls the callback function where we echo the HTML to display the page.
Parent Slug
In the above code, menu_title is Sos Settings and parent slug is options-general.php and this will show Sos Settings menu item under Settings menu. But, in the Share on Social Project code, the first argument of add_submenu_page() is ’edit.php?post_type=sos’ instead of ‘options-general.php’. This is because we want to list the sub menu item under a custom menu named Share on Social. We will cover the custom menu in a later chapter that deals with custom posts.
For the time being you can try out both slugs and see the placement of the sub menu.
At this point, when we click the new sub menu it displays a blank page. We will complete it soon. But before rendering the page, we need to register page fields with WordPress. In the next section, we go through WordPress Settings API and learn how to add sections and fields to a menu page.