12.2. WordPress Test Setup
In the previous blog, WordPress PHPUnit Setup we put up all the scaffolding necessary to run WordPress Plugin Tests. In this blog, we test the setup by running some test cases.
Run Test Cases
The test cases are placed in plugins’ tests/phpunit/tests
folder.
Let’s start with a test case to test the constants defined in the
wp-simple-plugin.php
. Add a test file test-wp-simple-plugin.php
to
tests/phpunit/tests
with following contents.
wp-simple-plugin/tests/phpunit/tests/test-wp-simple-plugin.php
<?php
class Test_WP_Simple_Plugin extends WP_UnitTestCase {
public function test_constants () {
$this->assertSame( 'wp-simple-plugin', WPSP_NAME );
$url = str_replace( 'tests/phpunit/tests/', '',
trailingslashit( plugin_dir_url( __FILE__ ) ) );
$this->assertSame( $url, WPSP_URL );
}
}
PHPUnit is an Object Oriented Testing Framework and we define test class
by extending the WordPress Tests Library class WP_UnitTestCase
. The
test methods are prefixed with test_ and in the test method
test_constants()
we assert whether constants WPSP_NAME and WPSP_URL
exists.
Let’s run test and see how it progresses.
$ cd wp-simple-plugin
$ phpunit
PHPUnit is called from the top directory of the plugin where it reads the phpunit.xml and executes test files defined by it.
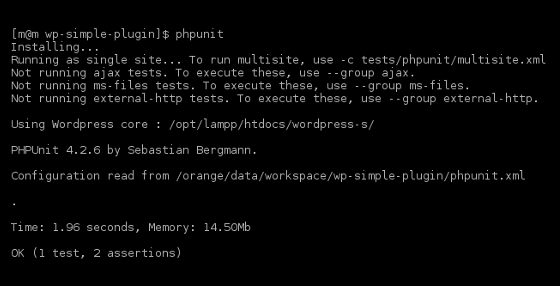
If all is well, then you should get see OK at the end of result with counts of tests and assertions.
Troubleshoot the Setup
But, many things tends to go wrong when we first execute the tests. Some of the typical errors and how to troubleshoot them are explained here.
Database Connection Error
When Test Lib is unable to connect to test database, it displays error message in HTML format. Resolve database connection error by going through following steps.
check whether you are able to connect to wptest database through mysql client by using username - wptest and password - wptest.
$ cd /opt/lampp $ bin/mysql --pass=wptest -u wptest wptest mysql> exit
In case, you are unable to connect to MySQL then check whether mysql is up and running by using ps in Linux or process manager in Windows.
next, check whether you are able to connect to MySQL through network layer by using host name and port.
$ cd /opt/lampp $ bin/mysql --pass=wptest -u wptest --host=localhost.localdomain --port=3306 wptest mysql> exit
In case, you are unable to connect to MySQL then check whether mysql is up and running and its listening port is 3306 by using ps in Linux.
Host Name
One more oddity we found was the host name and MySQL version. When path is pointed to MySQL version 5.3.x then we are able run tests with DB_HOST (wp-tests-config.php) is set as localhost.localdomain but not with localhost. However, with MySQL version 5.5.x we are able to run test even when DB_HOST is set as localhost.
WordPress Core Path Error
The ABSPATH definition in wp-tests-config.php should end with a trailing slash otherwise WordPress is unable to construct the absolute path of include files.
Permission Error
One more error is related to permission of WordPress directory.
1) Test_WP_Simple_Plugin::test_constants
UnexpectedValueException: RecursiveDirectoryIterator::__construct(/opt/lampp/wptest/wordpress/wp-content/uploads): failed to open dir: No such file or directory
The error indicates that WordPress instance created by Tests Library is
unable to access the uploads directory in wordpress/wp-content
. The
user who runs the test should have write permission on wp-contents
directory. To rectify this, either change the owner of the
/opt/lampp/wptest/wordpress
or add the user to group which owns the
the wordpress directory.
Plugin Link Error
When you run tests that checks the constants if you get following error then it means Test Lib is unable to load the plugin main file (and also the plugin) and because of that constant WPSP_NAME is not defined.
There was 1 failure:
1) Test_WP_Simple_Plugin::test_constants
Failed asserting that two strings are identical.
--- Expected
+++ Actual
@@ @@
-wp-simple-plugin
+WPSP_NAME
/orange/work/tem/wp-simple-plugin/tests/phpunit/tests/test-wp-simple-plugin.php:7
FAILURES!
Tests: 1, Assertions: 1, Failures: 1.
To rectify this, you need to create link in wordpress/wp-content/plugins directory pointing to the actual location of the plugin code as explained in Create Link to Plugin.
PHPUnit Error
XAMPP comes with PHPUnit and PHP. On one occasion, PHPUnit threw some dependency error which happened when /opt/lampp/bin was in users $PATH. However, subsequently the problem has not repeated. We have never faced this problem when we use stock PHP that comes with Linux and phpunit.phar installed as explained in the previous blog.
WordPress Options and Tests
In the previous blog, we used following code in
tests/phpunit/bootstrap.php
to activate and load the plugin.
wp-simple-plugin/tests/phpunit/bootstrap.php
$GLOBALS[ 'wp_tests_options' ] = array(
'active_plugins' => array(
'wp-simple-plugin/wp-simple-plugin.php'
),
'wpsp_test' => true
);
The array at index active_plugins is used by Tests Library to activate and load the plugin as part of WordPress initialization. The subsequent items in the array are used to create WordPress Options that are available during testing. With the above code, Tests Library creates an WordPress Option named wpsp_test and sets it value as true. Within test cases, we can refer this temporary option using WordPress Options API.
To test the option declared in bootstrap.php, add a new test case to the test file and re-run the phpunit.
wp-simple-plugin//tests/phpunit/tests/test-wp-simple-plugin.php
public function test_wpsp_option () {
$this->assertTrue(get_option('wpsp_test'));
}
In the next blog, we explain the various patterns used to unit test the Share on Social Plugin.