Maven Plugin Execution
In the previous tutorial, we saw plugin configuration. In this tutorial, we cover the Maven Plugin execution configuration.
Configure Plugin Execution
The element <executions>/<execution> allows you to configure the execution of a plugin goal. With it, you can accomplish the following things.
bind a plugin goal to a lifecycle phase.
configure plugin parameters of a specific goal.
configure plugin parameters of a specific goal such as compiler:compile, surefire:test etc., that are by default binds to a lifecycle phase
Let’s demonstrate plugin execution with an example. In Apache Ant, it’s quite easy to output echo any property or message during the build and many of us frequently use it to understand the build flow or to debug. But, Maven comes with no such feature, and only way to echo any message is to use Ant within Maven. The Apache AntRun Plugin provides the ability to run Ant tasks within Maven.
Let’s configure maven-antrun-plugin to output message to console.
simple-app/pom.xml
...
<build>
<plugins>
<plugin>
<artifactId>maven-antrun-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>run</goal>
</goals>
<phase>compile</phase>
<configuration>
<tasks>
<echo>Build Dir: ${project.build.directory}</echo>
</tasks>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
...
Build the project with mvn package
and it echoes the build directory
name in compile phase.
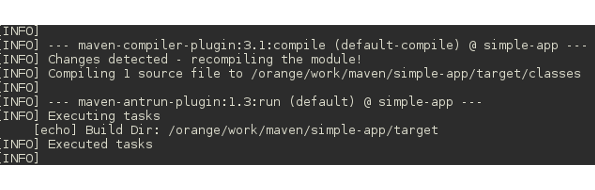
Let’s go through the above snippet to understand the execution. In the
<execution> element, we indicate that it should be applied to
antrun:run goal. We can apply an execution to one or more goals. Next,
we bind the execution to compile lifecycle phase and the antrun:run goal
binds to compile phase. Finally, we add <configuration> to the
execution. In configuration, we set task
and echo
parameters of
antrun:run goal to echo the build directory name.
In the above execution, we attached antrun:run goal to compile phase. But it can be any other phase and during execution of that phase ant will echo the output. Change the phase to test or verify phase and observe the output.
Maven Properties
In the configuration, we used a Maven property ${project.build.directory}. Maven properties are value placeholder, like properties in Ant and their values are accessible anywhere within a POM by using the notation ${X}, where X is the property.
Maven allows five types of properties - Environment variables, Project properties, properties defined in Settings files, Java System Properties and properties defined in POM. To know more about properties, refer Maven: The Complete Reference - Properties and POM Reference - Properties.
In the previous example, we saw that we can apply one or more goals to an execution. It is also possible to use one goal in multiple executions. Suppose, in the default jar, we want to exclude certain packages and create a separate jar with those packages. We can use multiple execution declarations to achieve this.
simple-app/pom.xml
...
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<executions>
<execution>
<id>default-jar</id>
<configuration>
<excludes>
<exclude>**/extrapackage/*</exclude>
</excludes>
</configuration>
</execution>
<execution>
<id>special-jar</id>
<phase>package</phase>
<goals>
<goal>jar</goal>
</goals>
<configuration>
<includes>
<include>**/extrapackage/*</include>
</includes>
<classifier>extra</classifier>
</configuration>
</execution>
</executions>
</plugin>
...
In execution with id default-jar we exclude some package and then add one more execution where jar:jar is again binds to package phase and in its configuration we include some package. The classifier parameter of jar:jar appends the string to jar name. The above snippet, generates two jars - simple-app-1.0.jar and simple-app-1.0-extra.jar.
In the next tutorial, we cover Project Object Model (POM) in detail.