Maven Profiles
Maven Profiles which allows to define multiple set of configurations and activate them based on certain conditions.
Maven Profile is an alternative set of configurations which set or override default values. Profiles allow to customize a build for different environments. Profiles are configured in the pom.xml and each profile is identified with an identifier.
Profiles customizes the build for different environments. Some of the examples of different environments are:
production and development systems.
platforms with different OS such as Linux, Window etc.,
different versions of JDK.
Let’s go through an example to learn Maven Profile. By default, Compiler Plugin sets Javac flags debug as true and optimize as false. For production code, we want to optimize the Java code and disable debugging info and to do that, we can use Maven profile.
From the Simple App pom.xml, remove the build declaration and add the following snippet.
simple-app/pom.xml
<!-- project build -->
...
<profiles>
<profile>
<id>production</id>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<debug>false</debug>
<optimize>true</optimize>
</configuration>
</plugin>
</plugins>
</build>
</profile>
</profiles>
...
We are defining a profile named production and place the build and plugin configuration within it. In the plugin configuration, we configure the Compiler Plugin’s parameters debug and optimize.
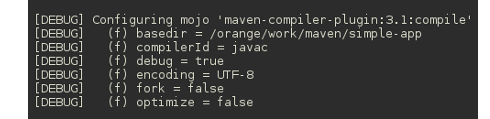
Build the project with command mvn compile -X
. The option -X
outputs Maven debug info to console and from the lengthy debug output,
the relevant part is shown in the screenshot. From the output, it is
clear that Javac debug is still true and optimize is false which
means that the production profile is not activated by Maven and it
is still using default configurations.
To activate the production profile use command mvn compile -Pproduction -X
. The option -P activates the profile. From the Maven debug
messages, we can confirm that profile is activated and Javac flags debug
and optimize are set as configured in the profile.
Profile Elements
By defining a profile we override the values defined by project’s Effective POM. Maven allows following elements in an Profile.
pom.xml
<project>
<profiles>
<profile>
<build>
<defaultGoal>...</defaultGoal>
<finalName>...</finalName>
<resources>...</resources>
<testResources>...</testResources>
<plugins>...</plugins>
</build>
<reporting>...</reporting>
<modules>...</modules>
<dependencies>...</dependencies>
<dependencyManagement>...</dependencyManagement>
<distributionManagement>...</distributionManagement>
<repositories>...</repositories>
<pluginRepositories>...</pluginRepositories>
<properties>...</properties>
</profile>
</profiles>
</project>
Virtually everything may be overridden by the profile. Maven Profile can override dependencies and the behavior of a build via plugin configuration. It can also override the configuration of distribution settings; for example, we can define a staging profile and use distributionManagement element to publish the artifacts to a staging repository.
Profile Activation
In the previous example, we activated the production profile by using option -P, but Maven provides other ways to activate the profiles.
Maven Profile can also be activated through profile/activation element based on state of build environment such as
JDK versions.
environment variables.
OS settings.
present or missing files.
Some of the profile activation examples are provided below.
pom.xml
<profiles>
<profile>
<id>dev</id>
<activation>
<activeByDefault>true</activeByDefault>
</activation>
...
</profile>
</profiles>
The above snippet, activates the dev profile by default.
pom.xml
<profile>
<id>include-script</id>
<activation>
<jdk>1.6</jdk>
</activation>
...
</profile>
If JDK version is 1.6, then profile include-script is activated.
pom.xml
<profile>
<id>win-build</id>
<activation>
<os>
<name>Windows XP</name>
<family>Windows</family>
<arch>x86</arch>
</os>
</activation>
...
</profile>
Profile win-build is activated when Maven builds on Windows XP system.
pom.xml
<profile>
<id>debug</id>
<activation>
<property>
<name>debug</name>
</property>
</activation>
...
</profile>
If property ${debug} is defined, then profile is activated.
pom.xml
<profile>
<activation>
<file>
<exists>target/generated-sources/foo</exists>
<missing>target/generated-sources/bar</missing>
</file>
</activation>
...
</profile>
Profile is activated if file foo exists and bar doesn’t exists in
target/generated-sources
directory.
For further information on Maven Profiles refer Introduction to Build Profiles and Maven Reference Book - Build Profiles.
In the next chapter, we look at Maven Multi Module Projects.