Chapter 5. Model
Domain Model
Data model of the application consists of following classes
Symbol – holds details about a symbol
DataGroup – holds various categories of data like BS (Balance Sheet), PL (Profit and Loss), CF (Cash Flow) etc
Data - segregates the data into different time periods.
Fact - holds key and value for each item of financial indicator
UML diagram of the model is as shown in figure
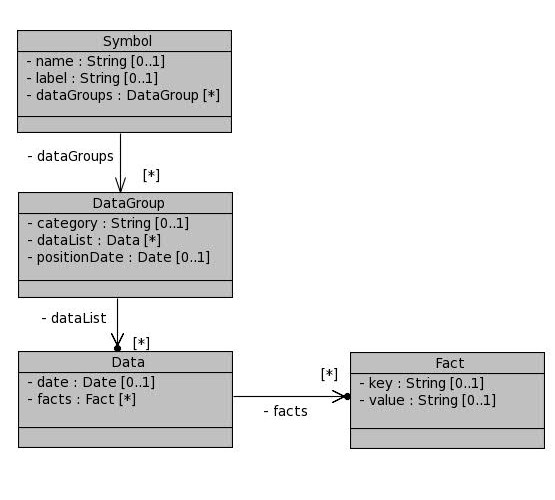
Figure 5.1. UML diagram of the Model
Model is explained with data of a imaginary company - Acme Ltd
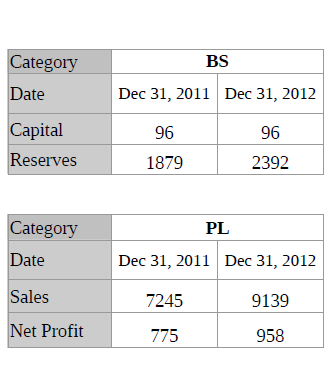
These data result in following domain objects.
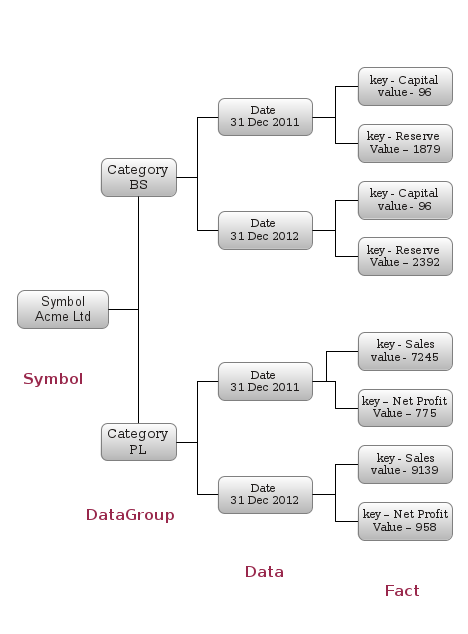
Figure 5.2. Example Data
Symbol holds name of company and a list of two DataGroup - one for category BS and another for PL.
Each DataGroup holds a list of two Data - one for 31 Dec 2011 and another for 31 Dec 2012
Each Data holds list of two Fact pertaining to a time period.
Each Fact contains a key and value.
Model classes
Model classes are in in.fins.client package. Apart from getters and setters they also provide some additional methods for basic operations
Import Fins Code
From this Chapter onwards the book will not have detailed listing of source, instead you have to use the sample code that accompany the book. Sample code is zipped into part-1.zip, part-2.zip etc., Use them for respective parts of the book and for each Chapter or Section, import sample code as follows.
Download the zip files from Fins Code tab of the site and unzip the archive to some location. Next, select Fins Project in Project Explorer and choose File → Import and in Select window expand General and select File System and click Next. In File System window, browse and select the location where you have extracted the archive. Extracted archive contains Chapter and Section folders and out of them, select the desired Chapter (ch- prefix) or Section ( sec- prefix) folder. Select src and war folders checkbox as shown in the next figure. In Into Folder field, enter fins. Check Overwrite existing resources, but deselect the Create top-level folder and click Finish. Now project has the source up to the end of that chapter or section.
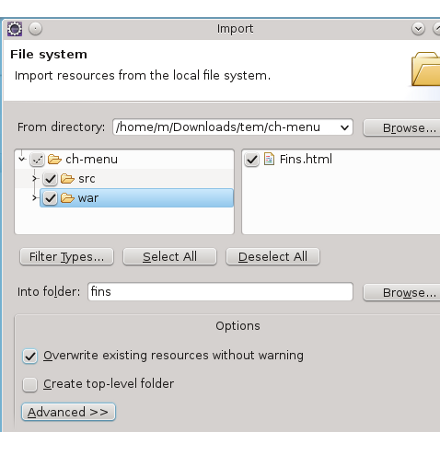
Figure 5.3. Sample selection
For many of us browsing full blow source code is bit tedious and hence incremental source code are placed into Chapter and Section folders in the zip. If you are comfortable in browsing the full blown source, then you free to select src and war from full-code folder which imports source as at end of that part of the book.
After code is imported, clean the project using Project → Clean
. This rebuilds all classes under war/WEB-INF/classes
. Project may
throw strange errors if old classes are lying around in that directory.
GWT compiler uses gwt-unitCache dir under project directory to speed-up the compile. After source import, delete gwt-unitCache dir else GWT may use earlier compilation units from cache. This step is only applicable when you import Fins code as file time-stamp may not be in proper order. No need to do this when you develop your own app except as a last resort.
Errors
Normally when you run the web app, builder takes care to compile the project using GWT compiler. But, even after cleanup if you still encounter runtime errors then you may try to compile the project yourself. Select project in Project Explorer view and invoke GDT Pull Down (Google Drop Down menu) from tool bar. Choose GWT Compile Project… option from pull down. GWT complier should compile without any errors.
In case error still lingers, then as a last resort, delete the in.fins
package (including all sub packages) from src directory to remove
everything from src dir. Next, do a fresh import of required
chapter/section, clean the project and do a GWT compile.
Symbol
addDataGroup(DataGroup)
- Add dataGroup to List<DataGroup>. Creates ArrayList if list is null.getDataGroup(String category)
- returns DataGroup for a category.
DataGroup
addData(Data)
- Add data to List<Data>. Creates ArrayList if list is null.getData(Date)
- returns Data for a date from dataList.firstDate()
- returns lowest date from dataList.lastDate()
- returns highest date from dataList.getDateList()
- returns sorted list of dates from dataList.
Data
addFact(Fact)
- Add fact to List<Fact>. Creates ArrayList if list is null.compareTo(Data)
- implementation of Comparable interface. Compares date field of Data with date field of data passed as argument.
SymbolHelper
Code access Symbol object to get other domain objects like DataGroup,
Data and Fact. These boiler plate codes are extracted into a utility
class SymbolHelper
with following static methods.
access methods
getDataGroup(Symbol, String category)
returns DataGroup of a categorygetData(Symbol, String category, Date)
returns Data of a category for a dategetFacts(Symbol, String category, Date)
returns List<Fact> for a category and date.getFact(Symbol, String category, Date, String key)
returns Fact for a date, category and key.getLastDate(Symbol, String category)
returns maximum Date for a category.getPositionDate(Symbol, String category)
returns Date for a category for which data is displayed.getOffsetDate(Symbol, String category, Date, int offset)
returns Date for a category which is date + offset. For example if symbol hold data for four dates 31 Mar 2011, 30 Jun 2011, Sep 2011 and 31 Dec 2011 then for date 30 Sep 2011 offset 1 returns 31 Dec 2011, offset -1 returns 30 Sep 2011, offset -2 returns 31 Mar 2011 and offset 0 returns 30 Sep 2011getCategories(Symbol)
returns List<String> with all category names which symbol is holding.
operations
addFact(Symbol, String category, Date, Fact fact)
add the Fact to Symbol. Fact is inserted to Data with specific Date of DataGroup of specific category.setPositionDate(Symbol, String category, Date)
set poistionDate to date for particular category.setFacts(Symbol, String category, Date, List<Fact>)
List<Fact> is set to particular Data of a DataGroup.updateSymbol(Symbol srcSymbol, Symbol dstSymbol)
copy data structure from srcSymbol to dstSymbolreplaceData(Symbol, String category, Data)
replace Symbol’s Data for a category with given Data.
SymbolDatabase
This part of book deals with client code, and therefore we are not making any call to the server. We need some data to display and in-memory data store in.fins.client.SymbolDatabase constructs and provides random data for symbols. It has private methods to construct data for various categories like BS, PL etc. It provides data to clients through two public methods.
List<String> getSymbolNames() - returns list of symbol names
Symbol getSymbol(String name,Map<String, String[]> filterMap) – for a given name returns a fully populated Symbol.
filterMap is a HashMap where key is category like BS, PL etc and value is array of String. With filterMap client indicates which Facts are required. For example if filterMap has key “Quote” and value { “Price”, “P/E” } then getSymbol() returns Symbol with facts for Price and P/E for Category Quote.
Data for following dates are returned for each category.
Table 5.1. Dates
Category | Date Frequency | Dates |
---|---|---|
Quote | Monthly | 31 Jan 2012, 29 Feb 2012 . . . . 31 Dec 2012 |
Holding | Monthly | 31 Jan 2012, 29 Feb 2012 . . . . 31 Dec 2012 |
Quarterly | Quarterly | 30 Sep 2011, 31 Dec 2011 . . . . 30 Sep 2012 |
BS | Yearly | 31 Dec 2007, 31 Dec 2008 . . . . 31 Dec 2012 |
PL | Yearly | 31 Dec 2007, 31 Dec 2008 . . . . 31 Dec 2012 |
Cash Flow | Yearly | 31 Dec 2007, 31 Dec 2008 . . . . 31 Dec 2012 |
For the previous example, getSymbol() returns Symbol with Facts for Price and P/E for category Quote for twelve periods i.e. Monthly from 31 Jan 2012 to 31 Dec 2012
Java Libraries
If you go through SymbolDatabase
you will notice couple of GWT
classes, com.google.gwt.user.client.Random
and
com.google.gwt.i18n.client.DateTimeFormat
. Java also offers Random
for generation of random numbers and SimpleDateFormat
to format date
from string, but we choose to use the equvilent GWT classes. Reason
being, client are allowed to use only libraries and Java language
constructs that are translatable to JavaScript and Java Random
and
SimpleDataFormat
are not translatable. It is important remember that
client side code written in Java is converted to JavaScript, and because
of difference between Java and Javascript GWT is not able convert many
of Java libraries to Javascript. To avoid runtime errors, you have to be
careful while selecting the Java library classes for client side coding.
Please refer Coding Basics - Compatibility with the Java Language and Libraries for more info.
Now that we have a simple in memory database which provides Symbols and its data to display on content page. Lets design first content page