7.2. WordPress Meta Box
In the previous blog, we saw how to register a new custom post type named sos and use it as Social Lockers. To manage the custom post type items, in this blog we setup, render and customize Sos Locker editor with WordPress Meta Box.
While registering the custom post type, we used
register_meta_box_cb to register the callback method
setup_meta_boxes()
.
share-on-social/admin/class-sos.php
public function create_post_type () {
register_post_type( $this->post_type,
// other args
'register_meta_box_cb' => array($this,'setup_meta_boxes')
) );
....
}
When we add or edit Sos Locker WordPress calls the callback, which we use to customize meta boxes of the editor.
WordPress Meta Box
WordPress Post editor is composed of Meta Boxes which holds HTML input and other elements. The screenshot of Sos Locker editor shows three meta boxes. The main meta box titled Share Locker is to edit lockers' meta data. On the right there are two meta boxes - CodeTab which is used to advertise this tutorial and the standard Publish meta box that WordPress adds to post editor.
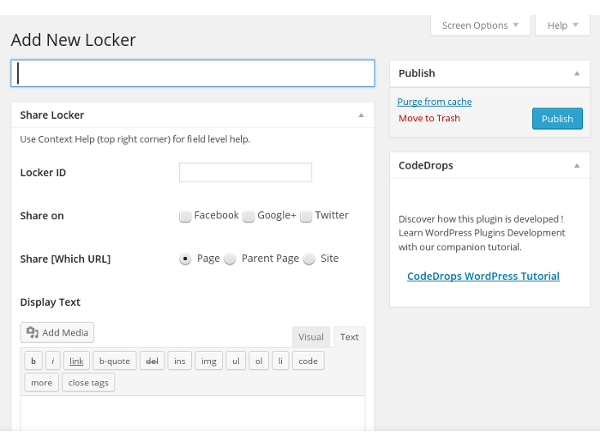
Let’s see how this is done. In the callback method setup_meta_boxes()
,
we use two WordPress functions - add_meta_box() and remove_meta_box().
share-on-social/admin/class-sos.php
function setup_meta_boxes ( $post ) {
add_meta_box( 'locker_meta_box', __( 'Share Locker', 'sos-domain' ),
array($this,'render_locker_meta_box'), $this->post_type, 'normal', 'high' );
add_meta_box( 'codedrops_meta_box', 'CodeDrops',
array($this,'render_codedrops_meta_box'), $this->post_type, 'side' );
remove_meta_box( 'slider_sectionid', $this->post_type, 'normal' );
remove_meta_box( 'layout_sectionid', $this->post_type, 'normal' );
}
To add Share Locker meta box, we use following arguments.
id - locker_meta_box,
title - Share Locker.
callback - method
render_locker_meta_box()
renders this meta box.post_type - only applicable to custom post type and we set it sos.
context - edit page is divided into three area - normal, advanced and side. We use normal to position the meta box below the title field. While, advanced places the meta box below the normal and side places box on the side bar.
priority - when there are multiple meta boxes in each area (context), their placement may fine tuned with priority - high, core, default and low.
callback_args - arguments to callback, if any.
We similarly add the second meta box to the side bar. WordPress adds other standard meta boxes - Layout and Slider - to the editor. Sos Lockers editor doesn’t require these two meta boxes. We use remove_meta_box() function to remove these meta boxes for sos custom post type.
Let’s get back to Share Locker meta box and see how it is rendered by the callback.
share-on-social/admin/class-sos.php
function render_locker_meta_box () {
wp_nonce_field( 'save_locker', 'locker_nonce' );
echo $this->get_table();
$this->add_editor();
}
function get_table () {
global $post;
$locker_id = get_post_meta( $post->ID, 'locker_id', true );
// other meta data
$locker_id_label = __( 'Locker ID', 'sos-domain' );
// other labels
$table = <<<EOD
<div>$see_help_message</div>
<table class="form-table">
<tr>
<th scope="row"><label for="locker_id">$locker_id_label</label></th>
<td><input type="text" name="locker_id" id="locker_id" value="$locker_id" /></td>
</tr>
// html elements of other metadata fields
</table>
EOD;
return $table;
}
In the rendering method, we set nonce token which is a security feature of WordPress to authenticate the requests. Next, we echo a HTML table containing the the required text fields, check boxes and radio buttons.
When we edit a locker, WordPress sets the locker object which is a sos
custom post of type in the global variable $post and we use the $post
object to get the post_id of the locker as $post->ID
.
Locker edit page also has TINY MCE Visual Editor at the bottom to enter
the display text of the locker. Rendering method calls add_editor()
method to add the TINY MCE editor.
share-on-social/admin/class-sos.php
function add_editor () {
global $post;
$id = 'locker_text';
$name = 'locker_text';
$value = wp_kses_post( get_post_meta( $post->ID, 'locker_text', true ) );
$editor_options = array(
'textarea_name' => $name,
'textarea_rows' => 10,
'tinymce' => array(
'toolbar1' => 'bold italic underline | alignleft aligncenter alignright | outdent indent | undo redo | fullscreen',
'toolbar2' => 'formatselect fontselect fontsizeselect | forecolor backcolor | removeformat'
)
);
wp_editor( $value, $id, $editor_options );
}
In add_editor() method, we use TINY MCE editor options to set essential toolbar icons and then call WordPress wp_editor() function which adds the visual editor. When post is saved we can fetch the text entered in editor from the $_POST[’locker_text’] as the editor option textarea_name is set to locker_text.
Post Contents
Share on Social plugin saves locker display text as meta data. The Display Text field is just a bunch of HTML text and it may very well saved as post content instead of separate meta data.
While registering sos custom post type, we disabled the default content editor that WordPress adds to the post editor and enabled a separate TINY MCE editor to enter the display text. We do so to have a better control over the editor. WordPress places the default content editor at the top of the page. As it is meant to edit post content, its dimension is quite large. By going for custom TINY MCE editor, we could do the following.
place the editor at the bottom of meta box after all other fields.
make editor smaller.
disable some of the toolbar items.
Hide Publish Details
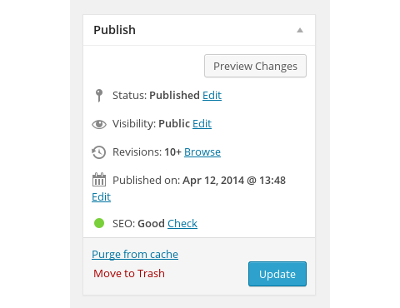
The default Publish Meta box has editable fields such as Status,
Visibility and Published on etc., Sos Lockers doesn’t require
these information. The block that displays these things is the
minor-publishing section and there is no documented way to remove it.
Instead, we toggle the block style and hide it in hide_minor_publish()
method which is hooked to admin_head hook.
share-on-social/admin/class-sos.php
function hide_minor_publish () {
$screen = get_current_screen();
if ( in_array( $screen->id,
array($this->post_type) ) ) {
echo '<style>#minor-publishing { display: none; }</style>';
}
}
In the next section, we see how to publish the post.