In the tutorial, so far, we covered WordPress Plugin basics and the admin module. In this chapter, we describe the frontend of Share on Social Plugin, the plugin project developed in the tutorial.
For frontend, Share on Social Plugin uses class file
class-frontend.php
and the methods may broadly divided into three
groups.
which handle locker shortcode, fetch locker information and output social lockers.
which handle scripts and stylesheets.
methods to collect debug info.
We will not dwell much into the first one as you may not develop a similar plugin. Instead, we explain the other two - handling of scripts and collect debug info - as they are essential to all plugins.
8.1. WordPress Plugin Enqueue Scripts
Share on Social Plugin uses following scripts and stylesheets.
Stylesheet of the plugin. - css/style.js.
JavaScript to handle social shares - js/share.js.
FaceBook API JavaScript- https://connect.facebook.net/en_US/all.js
Google Plus API JavaScript - https://apis.google.com/js/plusone.js
Twitter API JavaScript - https://platform.twitter.com/widgets.js
To add these scripts and stylesheet, either to header or footer, we have to use WordPress API Enqueue Functions.
- registers a script with WordPress core.
- links script file to WordPress generated page.
- registers stylesheet.
- links stylesheet to WordPress generated page.
In Sos_Frontend::setup() method, we hook add_sos_script()
method to
action hook wp_enqueue_scripts. In the method, we enqueue scripts
and stylesheets.
share-on-social/frontend/class-frontend.php
class Sos_Frontend {
public function setup () {
add_action( 'wp_enqueue_scripts', array($this,'add_sos_scripts') );
....
}
function add_sos_scripts () {
global $post;
wp_register_style( 'sos_style', SOS_URL . '/css/style.css' );
wp_register_script('sos_script', SOS_URL . '/js/share.js', array('jquery'),'', true);
if ( has_shortcode( $post->post_content, $this->sos_shortcode ) ) {
wp_enqueue_style( 'sos_style' );
wp_enqueue_script( 'sos_script' );
....
$twitter_sdk = 'https://platform.twitter.com/widgets.js';
wp_enqueue_script( 'twitter', $twitter_sdk, '', '', FALSE );
....
}
}
In the action method, we register plugins stylesheet css/style.css
and
script js/share.js
. If the page contains share-on-social
shortcode, then we enqueue the registered items and also the Twitter API
script.
The script enqueue functions wp_register_script() and wp_enqueue_script() takes following parameters.
handle - name used as handle to the script.
src - URL of the script.
deps - array of handles of the registered scripts that this script depends on.
ver - string to specify the script version number.
in_footer - if true, then script is added to footer.
These functions links script files at the right time according to the
script dependencies. While registering js/share.js, we passed array( 'jquey')
in the third parameter - deps. It indicates that our script
depends on JQuery script and WordPress links share.js after JQuery
script is added to the page.
Version parameter adds version number to end of URL as query string ?ver=x.x. It is used to ensure that the correct version is sent to the client regardless of caching. To stop adding version to URL, set this parameter to null.
Enqueue functions, normally, adds script to the page <header>. If last parameter in_footer is set as true, then script adds to the <footer> provided the site theme has wp_footer() template tag at right place.
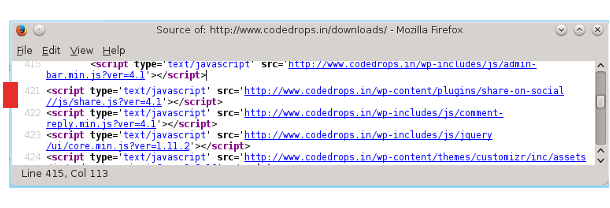
Register and Enqueue
We can enqueue script by calling wp_enqueue_script() without
pre-registering. In the above code, we may directly enqueue js/share.js
without registering it.
The function wp_register_script() is useful either to register all the scripts in one place and enqueue them when needed or to handle dependencies.
Scripts that are registered do not need to be manually enqueued, if they are listed as a dependency of another script that is enqueued. WordPress automatically links the registered script before it links the enqueued script that lists the registered script’s handle as a dependency. For example, say bar.js is depends on foo.js and foo.js is registered, then enqueue bar.js links both foo.js and bar.js, in the order, to the page.
Stylesheet register or enqueue functions are similar to script functions, except that stylesheets are always linked in <header> so there is in_footer parameter. Instead style functions has parameter - media, which specifying the media for which the stylesheet is defined.
The functions referred here are only for use with frontend and there are equivalent functions for admin screen and login screen.
WordPress jQuery
jQuery library included with WordPress is set to noConflict mode and in that mode, we are allowed to use jQuery(#somefunction) and not the normal jQuery shorthand $(#somefunction). For more information on this refer jQuery noConflict Wrappers
In the next section, we explain the steps to pass data to the client i.e. browser. We touch upon enqueue of Facebook and Google Plus scripts while doing that.