8.2. WordPress Pass Data to JavaScript
In the previous blog, we saw how to enqueue plugin scripts and stylesheets. We also enqueued an external JavaScript - Twitter API script. In this tutorial, we explain the steps to pass data to JavaScript.
WordPress Pass Data to Scripts
Share on Social Plugin can share the blogs to Facebook, Google Plus and Twitter. Out of the three, Twitter API is the easiest as it doesn’t require an App, while Facebook SDK requires an APP ID and Google Plus SDK expects a Client ID to share the blogs.
For Facebook, we can add the APP ID as a query string directly to the script URL as shown in the next code snippet.
share-on-social/frontend/class-frontend.php
function add_sos_scripts () {
....
if ( has_shortcode( $post->post_content, $this->sos_shortcode ) ) {
....
if ( false == empty( $options[] 'fbappid' ] ) ) {
$fb_app_id = $options[ 'fbappid' ];
$fb_sdk = "http://connect.facebook.net/en_US/all.js#xfbml=1&appId=$fb_app_id&version=v2.0";
wp_enqueue_script( 'sos_script_fb', $fb_sdk, array('jquery'), '', false );
}
....
}
}
However, for Google Plus we have to pass the Client ID to the Google Plus API function and to do that, we have to pass the Client ID to the plugin script share.js. To pass data to client and attach it to the enqueued JavaScript, WordPress provides function - wp_localize_script().
share-on-social/frontend/class-frontend.php
function add_sos_scripts () {
....
if ( has_shortcode( $post->post_content, $this->sos_shortcode ) ) {
....
$gplus_client_id = "";
if ( false == empty( $options[ 'gplusclientid' ] ) ) {
$gplus_client_id = $options[ 'gplusclientid' ];
$gplus_sdk = 'https://apis.google.com/js/plusone.js';
wp_enqueue_script( 'sos_script_gplus', $gplus_sdk, array('jquery'), '', false );
}
wp_localize_script( 'sos_script', 'sos_data',
array(
'ajax_url' => admin_url( 'admin-ajax.php' ),
'nonce' => wp_create_nonce( 'sos-save-stat' ),
'gplus_client_id' => $gplus_client_id
) );
}
}
Google Plus SDK doesn’t accept Client ID in the script URL. So, we use wp_localize_script() and pass data with it. The first parameter is script handle name to which the data belongs to and we attach data to sos_script which we enqueued in previous chapter. The second parameter is name of the data variable and third one is an array that holds the data. We use array to pass to three data items - ajax_url, nonce and gplus_client_id. Out of them, the first two - ajax_url and nonce - are related to ajax, which we cover in a later chapter.
The function creates a JavaScript block and converts the data array as JSON and assigns it to a variable named sos_data. WordPress adds the script block to the generated page and places the block just before the enqueue script as shown in the screenshot.
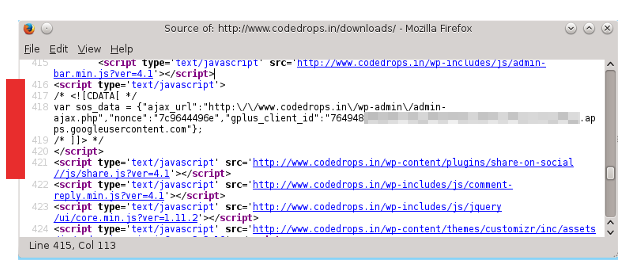
In the script, we can refer the passed data as shown in the next snippet.
share-on-social/js/share.js
function getGPlusShareOptions(shareName, shareTarget) {
var shareOptions = {
contenturl : shareTarget,
clientid : sos_data.gplus_client_id,
cookiepolicy : 'single_host_origin',
calltoactionlabel : 'DISCOVER',
calltoactionurl : shareTarget,
onshare : function(response) {
handleGPlusResponse(shareName, response);
}
};
return shareOptions;
}
In the script, we obtain Google Plus Client ID from the passed data with
sos_data.gplus_client_id
.
In the next section, we show how to collect debug info and output it, as comments, to the browser.