In this chapter, we step through the plugins’ main file. We also explain the benefits of PHP Classes to code the plugin instead of functions.
Plugin Info
The main file share-on-social.php
starts with header comments. As
already described in the “WordPress Simple Plugin”, WordPress uses the header comments
from the main file to display the Plugin info in plugins page.
Define Constants
Throughout the plugin, we often refer some paths and constants. Normally, the constants are defined at the start of plugins. In Share on Social, we define following constants.
share-on-social/share-on-social.php
define( 'SOS_NAME', 'share-on-social' );
define( "SOS_VERSION", '1.0.0' );
define( "SOS_URL", trailingslashit( plugin_dir_url( __FILE__ ) ) );
// plugin path
define( "SOS_PATH", plugin_dir_path( __FILE__ ) );
//basename share-on-social/share-on-social.php
define( "SOS_PLUGIN_BASENAME", plugin_basename( __FILE__ ) );
// main file - share-on-social.php
define( "SOS_PLUGIN_FILE", __FILE__ );
We prefix constants with SOS so that names don’t clash with constants from theme and other plugins. Always prefix the constants with the plugin name or some unique abbreviation.
Functions and Classes
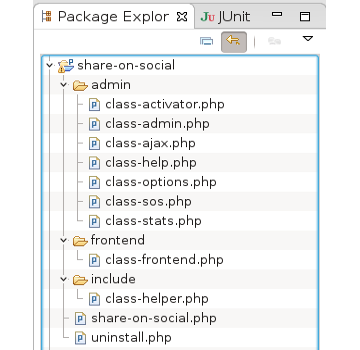
It is easy to code the plugin functionality with PHP functions. But this approach may results in name conflict either with functions defined by site theme and other active plugins or even with WordPress API.
Even though bit difficult to read, better approach is to use PHP Classes which eliminates the name conflict.
In Share on Social Plugin, we use classes for all modules except the main file and uninstall.php where we use regular functions. Rest of the code is split into class files which are included in main file.
Let’s see how this is done with two class files; one for front end and another for admin module. In the following code snippet, we include two class files class-frontend.php and class-admin.php.
share-on-social/share-on-social.php
// plugin entry point
setup_sos_plugin();
function setup_sos_plugin () {
require_once SOS_PATH . 'include/class-helper.php';
if ( is_admin() ) {
require_once SOS_PATH . 'admin/class-admin.php';
add_action( 'plugins_loaded', 'load_sos_admin' );
} else {
require_once SOS_PATH . 'frontend/class-frontend.php';
add_action( 'plugins_loaded', 'load_sos_frontend' );
}
}
function load_sos_admin () {
$sos_admin = new Sos_Admin();
$sos_admin->setup();
}
function load_sos_frontend () {
$sos_frontend = new Sos_Frontend();
$sos_frontend->setup();
}
in the main file, we call a single function
setup_sos_plugin()
which is also the the entry point of the plugin.in the function, we check whether we are in admin screen or in site with the help of
is_admin()
. WordPress function is_admin() return true when we are in the admin screen. Please note that we should not use this function to check whether the user has admin privileges or not.if we are in admin screen, then include
class-admin.php
and attachload_sos_admin()
function to WordPress hook plugins_loaded usingadd_action()
. Next chapter covers add_action() in depth, but for the time it is suffice to note that when all active plugins are loaded, WordPress calls our function load_sos_admin().in
load_sos_admin()
function, we create a object of classSos_Admin
and call itssetup()
method.when is_admin is false, we include class-frontend.php and load its class.
It is important to note that load_sos_admin()
and
load_sos_frontend()
are not called immediately by add_action() in
setup_sos_plugin()
, but their execution is deferred.
Just for the sake of explanation, we split this into two phases. When we
access the site, as part of phase one, WordPress processes all activated
plugins. When share-on-social.php
is processed its
setup_sos_plugin()
function is called. In this function, WordPress
includes the class files and calls add_action() which just registers
load_sos_admin()
or load_sos_frontend()
as callback functions
with WordPress for a hook named plugins_loaded. Then WordPress goes
on to process other plugins which are active in the site. When all
active plugins are processed, WordPress triggers second phase by calling
the hook plugins_loaded. At this point, WordPress calls registered
callback methods load_sos_admin()
or load_sos_frontend()
and
executes the code they contain.
Next, let’s go through a plugin class file to see how classes are defined with a snippet from class-frontend.php .
share-on-social/frontend/class-frontend.php
<?php
defined( 'ABSPATH' ) or die( "Access denied !" );
class Sos_Frontend {
var $sos_shortcode = 'share-on-social';
public function setup () {
// enable shortcode
add_shortcode( $this->sos_shortcode,
array(
$this,
'enable_sos_shortcode'
) );
}
// other methods of the class
}
In the class file, we define class Sos_Frontend
and its method. At the
top, we define variables which have class scope such as
$sos_shortcode
. In classes, we use setup()
to carry out
initializations tasks such as add actions, filters, shortcodes and also
to include other class files and modules etc., Other class files of the
plugin follow similar pattern. For the moment, it is enough to
understand the overall structure of the plugin and its files as
throughout the tutorial, we cover its code in detail.
In the next chapter, we explain the the important WordPress concepts Actions, Filters and Hooks which allows plugins to plug to the WordPress core.