2.2. WordPress Shortcode
Let’s add some functionality to our plugin using WordPress Shortcode API.
This is our first brush with WordPress API and we go with Shortcode because they are easy to understand and implement.
WordPress Shortcode are macros that can be placed in posts. When processing the posts, WordPress replaces the shortcode with contents returned by a handler function.
Add following code to wp-simple-plugin.php
after defined( 'ABSPATH' ) or die...
line.
wp-simple-plugin/wp-simple-plugin.php
add_shortcode( 'our-shortcode', our_shortcode_handler );
function our_shortcode_handler ( $atts, $content ) {
return $content .
"<font style='font-size:24px; color: #a61f38;'>Added by our-shortcode!</font>";
}
We use WordPress function add_shortcode() to add a shortcode our-shortcode and attach a handler function our_shortcode_handler()
to it. Now, we can place shortcode
[our-shortcode]
some content ...
[/our-shortcode]
on any post or page. Post shows the content between the shortcode appended with a message.
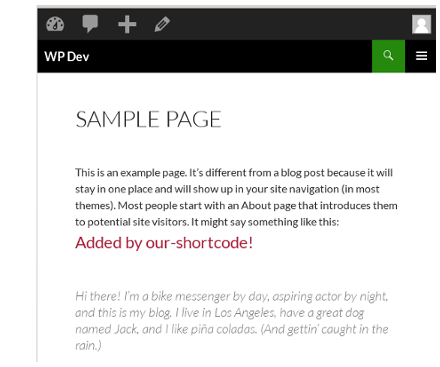
When WordPress processes the post it encounters the shortcode and calls the handler function and passes the content between the shortcode to the handler. In the handler, we append a message to the content and return it. WordPress replaces the shortcode and its contents with string returned by handler and displays the post.
We can also add attributes to a shortcode as follows.
[our-shortcode message='Hello' append='yes']
some content
[/our-shortcode]
WordPress passes attributes in an array to the handler function using $atts parameter.
WordPress Function Reference.
During plugin development, we frequently refer to functions provided by WordPress core. WordPress Functions Reference categories all functions based on its functionality. The version of the same is WordPress Code Reference
Keep them handy to explore the WordPress API while developing the plugin.
Hide the contents
In Share on Social plugin, we use shortcode share-on-social to hide the content and display the locker. We will explain the complete details in a later chapter and for now just show how to hide the content using shortcode.
Add following code to wp-simple-plugin.php.
wp-simple-plugin/wp-simple-plugin.php
add_shortcode('share-on-social', hide_content_handler);
function hide_content_handler ( $atts, $content ) {
$html = "<div style='display: none;'>" . $content .
"</div>";
return $html;
}
This code adds another shortcode and its handler. In the handler, we wrap the content in a <div> element and set its style to hide the block. This ensures that our shortcode is SEO friendly as underlying content is passed to browser so that search engines can crawl the page and index it properly.
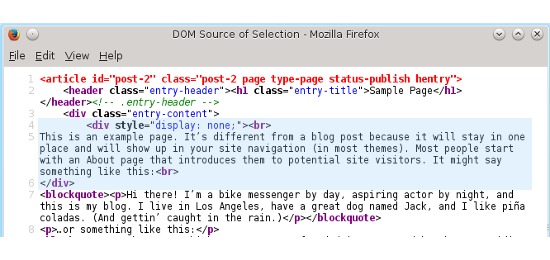
Even though contents between the shortcode are not displayed in browser, we can still find it when we view the source of the page.
So far, we simply add all the code to the main plugin page. This will soon mess up our plugin make it difficult to maintain. In a production WordPress Plugin, we need to structure the layout of the plugin in such a way that it is modular, tidy and also easy to test.
In the next section, we cover some of these aspects and explain how to modularize the plugin.